matplotlib ライブラリーのメソッドを使って、一つの描画キャンパスを複数の領域に分割して、それぞれのサブ領域にグラフを作成することができる。subplot メソッドを使うと、描画キャンパスを均等に分けられるのに対して、subplot2grid
メソッドを使うと、描画キャンパスを任意の割合で分けることができる。
subplot2grid
には最小限 2 つの引数を与える必要がある。1 番目の引数には画面を何行何列に均等に分けるのかをタプルの形で指定する。続いて、2 番目の引数には、これから描くグラフは、分割した領域のうち、どのサブ領域にグラフを描くのかをタプルの形で指定する。また、複数のサブ領域を結合したいときに colspan
または rowspan
を使用する。例えば、3 列をまとめて 1 つのサブ領域としたい場合は、colspan=3
のように指定する。
3 分割
import pandas as pd
import numpy as np
from matplotlib import pyplot as plt
import seaborn as sns
plt.style.use('default')
sns.set()
sns.set_style('whitegrid')
sns.set_palette('gray')
np.random.seed(2018)
# top left
x1 = np.random.uniform(0, 100, 20)
y1 = x1 * np.random.uniform(1, 2, 20)
plt.subplot2grid((2, 2), (0, 0))
plt.scatter(x1, y1)
plt.xlabel("weight [g]")
plt.ylabel("length [cm]")
plt.ylim(0, 190)
plt.xlim(0, 110)
# top right
x2 = np.array(['ERS1', 'ERS2', 'ETR1', 'ETR2', 'EIN4'])
y2 = np.array([12.0, 3.1, 11.8, 2.9, 6.2])
x2_position = np.arange(len(x2))
plt.subplot2grid((2, 2), (0, 1))
plt.bar(x2_position, y2, tick_label=x2)
plt.xlabel("gene")
plt.ylabel("expression [log(TPM)]")
# bottom left
x3 = np.random.normal(0, 1, 100)
plt.subplot2grid((2, 2), (1, 0), colspan=2)
plt.hist(x3, bins=20)
plt.xlabel("length [cm]")
plt.ylabel("frequency")
# show plots
plt.tight_layout()
plt.show()
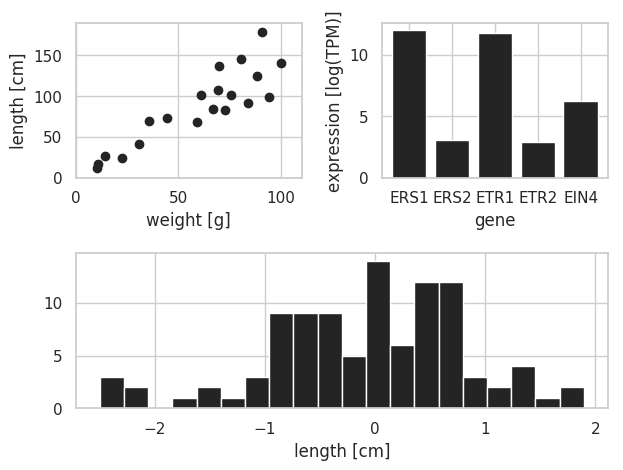
import pandas as pd
import numpy as np
from matplotlib import pyplot as plt
import seaborn as sns
plt.style.use('default')
sns.set()
sns.set_style('whitegrid')
sns.set_palette('gray')
np.random.seed(2018)
# top left
x1 = np.random.uniform(0, 100, 20)
y1 = x1 * np.random.uniform(1, 2, 20)
plt.subplot2grid((2, 2), (0, 0), rowspan=2)
plt.scatter(x1, y1)
plt.xlabel("weight [g]")
plt.ylabel("length [cm]")
plt.ylim(0, 190)
plt.xlim(0, 110)
# top right
x2 = np.array(['ERS1', 'ERS2', 'ETR1', 'ETR2', 'EIN4'])
y2 = np.array([12.0, 3.1, 11.8, 2.9, 6.2])
x2_position = np.arange(len(x2))
plt.subplot2grid((2, 2), (0, 1))
plt.bar(x2_position, y2, tick_label=x2)
plt.xlabel("gene")
plt.ylabel("expression [log(TPM)]")
# bottom left
x3 = np.random.normal(0, 1, 100)
plt.subplot2grid((2, 2), (1, 1))
plt.hist(x3, bins=20)
plt.xlabel("length [cm]")
plt.ylabel("frequency")
# show plots
plt.tight_layout()
plt.show()
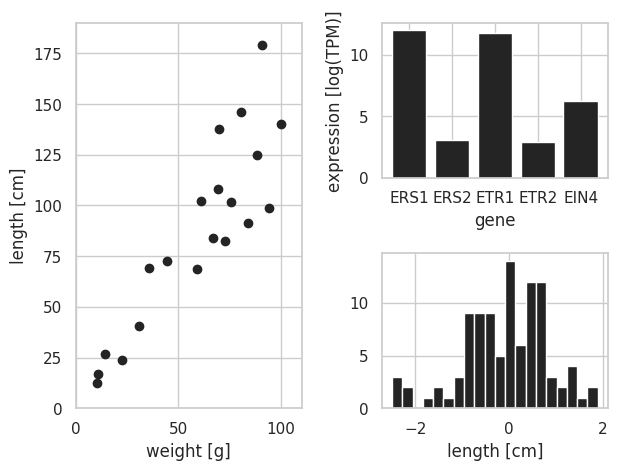
4 分割
import pandas as pd
import numpy as np
from matplotlib import pyplot as plt
import seaborn as sns
plt.style.use('default')
sns.set()
sns.set_style('whitegrid')
sns.set_palette('gray')
np.random.seed(2018)
# top left
x1 = np.random.uniform(0, 100, 20)
y1 = x1 * np.random.uniform(1, 2, 20)
plt.subplot2grid((2, 10), (0, 0), colspan=4, rowspan=1)
plt.scatter(x1, y1)
plt.xlabel("weight [g]")
plt.ylabel("length [cm]")
plt.ylim(0, 190)
plt.xlim(0, 110)
# top right
x2 = np.array(['ERS1', 'ERS2', 'ETR1', 'ETR2', 'EIN4'])
y2 = np.array([12.0, 3.1, 11.8, 2.9, 6.2])
x2_position = np.arange(len(x2))
plt.subplot2grid((2, 10), (0, 5), colspan=5, rowspan=1)
plt.bar(x2_position, y2, tick_label=x2)
plt.xlabel("gene")
plt.ylabel("expression [log(TPM)]")
# bottom left
x3 = np.random.normal(0, 1, 100)
plt.subplot2grid((2, 10), (1, 0), colspan=6, rowspan=1)
plt.hist(x3, bins=20)
plt.xlabel("length [cm]")
plt.ylabel("frenquency")
# bottom right
x4 = [0, 1, 2, 3, 4, 5]
y4 = np.array([11, 23, 27, 32, 18, 5])
plt.subplot2grid((2, 10), (1, 7), colspan=3, rowspan=1)
plt.plot(x4, y4)
plt.xlabel("time [hour]")
plt.ylabel("gene expression [log(TPM)]")
# show plots
plt.tight_layout()
plt.show()
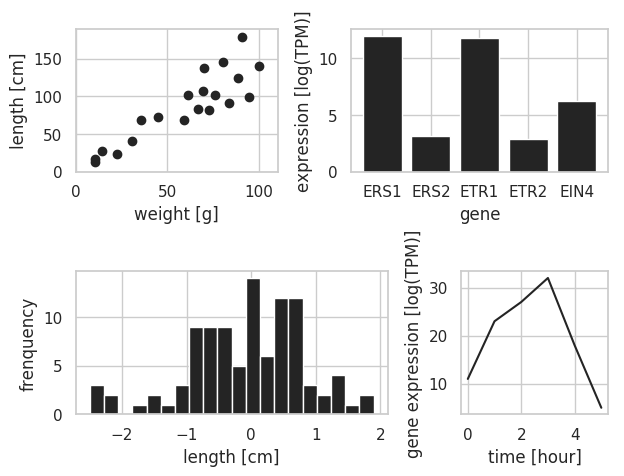